Язык Visual Basic на платформе .NET.
Модераторы: Ramzes, Sebas
-
winnydows
-
- Начинающий
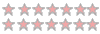
-
-
- Сообщения: 7
- Зарегистрирован: 12.02.2006 (Вс) 2:38
winnydows » 15.04.2006 (Сб) 23:00
Подскажите пожалуйста как управлять BeSweet.dll в среде Visual Basic 8. Есть пример на с++, но он для меня малопонятен. Может кто сможет ковертировать пример или знает как вызвать функции из этой DLL. Если к то не в курсе это библиотека обработки звука.
- Код: Выделить всё
// Example usage of BeSweet.dll by DSPguru. made April 2002.
// for the latest releases by Dg, visit : http://DSPguru.doom9.org
#include <windows.h>
#include <stdio.h>
#include <math.h>
#include "example2.h"
int main(int argc,char **argv)
{
char* ARGV[25]={"deinit","-core(","-input","ac3input","-output","test.mp3","-logfile ","BeSweet.txt",")","-ota(","-d","0","-G","max",")","-lame(","--alt-preset","128","--scale","1",")","-ssrc(","--rate","44100",")"};
int ARGC=21; // 25
/* here is the command line :
// dvd2avi.exe -core( -input ac3input -output test.mp3 -logfile BeSweet.txt )
// -ota( -d 0 -G max )
// -lame( --alt-preset 128 --scale 1 )
// -ssrc( --rate 44100 )
*/
/* explenation :
// "-input ac3input" helps BeSweet.dll to ignore the fact there is no input file
// "-logfile BeSweet.txt" will create a logfile, with info that can be displayed on dvd2avi
// in order to append to the logfile, write ARGV[6][8]='a'.
// "-d 0" sets no delay. to assert the A/V delay, change ARGV[11]
// "-G max" will assert the maximum gain whenever BeSweet is deinited.
// with this switch, you only need to supply the AC3 bursts once
// "--scale 1" : since we use the PostGain engine, we don't want lame to assert its own gain
// "--rate 44100" : to downsample the track, simply change ARGC to 25
*/
// BeSweet.dll win32 stuff
HINSTANCE hDLL =NULL;
BESWEET BeSweet =NULL;
BURSTS AC3_Bursts =NULL;
BURSTS MPA_Bursts =NULL;
VERSION BS_VERSION =NULL;
char buffer[2000]; // AC3 bursts
FILE* inputfile; // inputfile
int count=1; // burst size
BS_Record info; // BeSweet info
// load BeSweet.dll and its functions
hDLL=LoadLibrary("BeSweet.dll");
BeSweet = (BESWEET) GetProcAddress(hDLL, "BeSweet");
AC3_Bursts = (BURSTS) GetProcAddress(hDLL, "AC3_Bursts");
MPA_Bursts = (BURSTS) GetProcAddress(hDLL, "MPA_Bursts");
BS_VERSION = (VERSION) GetProcAddress(hDLL, "BS_VERSION");
switch (argc)
{
case 1:
fprintf(stderr,"Usage : dvd2avi inputfile [outputfile] [logfile] [delay value] [use ssrc] [append to logfile]\n");
exit(-1);
case 7:
ARGV[6][8]='a'; // append to logfile
case 6:
ARGC=25; // use ssrc
case 5:
ARGV[11]=argv[4]; // delay value
case 4:
ARGV[7]=argv[3]; // logfile
case 3:
ARGV[5]=argv[2]; // output filename
case 2:
inputfile=fopen(argv[1],"rb"); // open inputfile
}
fprintf(stderr,"Using %s\n",BS_VERSION());
// init BeSweet
*ARGV=*argv;
BeSweet(ARGC,ARGV);
/////////////////////////// Encoding loop ////////////////////////
// BeSweet.dll gets AC3 bursts, and create mp3 frames into file //
// Start Transcoding
while(count)
{
count=fread(buffer,1,2000,inputfile);
info=AC3_Bursts(count,buffer);
if (!info->err)
{ // print timestamp & maximum gain (this is a shared pass for both)
fprintf(stdout,"[%02d:%02d:%02d:%03d] transcoding! Max gain : %2.1fdB \r",info->hours,info->minutes,info->seconds,info->millis,-20*log10(info->peak));
}
}
//////////////////////////// Deinit BeSweet /////////////////////
// BeSweet.dll will close the file, and assert maximum gain
// deinit BeSweet
strcpy(ARGV[0],"deinit"); // this tells BeSweet.dll to deinit
BeSweet(ARGC,ARGV);
FreeLibrary(hDLL);
}
http://besweet.notrace.dk/BeSweetv1.5b23DLL.zip
-
minotawr
-
- Продвинутый пользователь
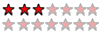
-

-
- Сообщения: 195
- Зарегистрирован: 08.01.2006 (Вс) 12:38
- Откуда: Курск
-
minotawr » 17.04.2006 (Пн) 7:53
Хм, также как и из любой другой ActiveX.
Подключаешь ее к проекту и юзаешь, броузер объектов поможет разобратся что к чему.
-
winnydows
-
- Начинающий
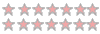
-
-
- Сообщения: 7
- Зарегистрирован: 12.02.2006 (Вс) 2:38
winnydows » 17.04.2006 (Пн) 11:31
@minotawr
Сложность в том что это не ActiveX, это Native DLL. Нужно делать экспорт функций, но вот как именно. Например к MediaInfo.dll это делается так:
- Код: Выделить всё
Private Declare Unicode Function MediaInfo_New Lib "apps\MediaInfo\MediaInfo.DLL" () As Integer
Private Declare Unicode Sub MediaInfo_Delete Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer)
Private Declare Unicode Function MediaInfo_Open Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal File As String) As Integer
Private Declare Unicode Sub MediaInfo_Close Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer)
Private Declare Unicode Function MediaInfo_Inform Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal Reserved As Integer) As String
Private Declare Unicode Function MediaInfo_Option Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal Optionn As String, ByVal Value As String) As String
Private Declare Unicode Function MediaInfo_GetI Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal StreamKind As Integer, ByVal StreamNumber As Integer, ByVal Parameter As Integer, ByVal KindOfInfo As Integer) As String 'See MediaInfoDLL.h for enumeration values
Private Declare Unicode Function MediaInfo_Get Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal StreamKind As Integer, ByVal StreamNumber As Integer, ByVal Parameter As String, ByVal KindOfInfo As Integer, ByVal KindOfSearch As String) As String
Private Declare Unicode Function MediaInfo_State_Get Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer) As Integer 'see MediaInfo.h for details
Private Declare Unicode Function MediaInfo_Count_Get Lib "apps\MediaInfo\MediaInfo.DLL" (ByVal Handle As Integer, ByVal StreamKind As Integer, ByVal StreamNumber As Integer) As Integer 'see MediaInfoDLL.h for enumeration values
Dim Aspect As String
Sub GetAspect()
Dim Handle As Object
Handle = MediaInfo_New()
MediaInfo_Open(Handle, file)
Aspect = MediaInfo_GetI(Handle, 1, 0, 20, 1)
MediaInfo_Close(Handle)
MediaInfo_Delete(Handle)
End Sub
-
Dmitriy Solomnikov
-
- Постоялец
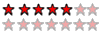
-
-
- Сообщения: 407
- Зарегистрирован: 10.11.2004 (Ср) 13:04
- Откуда: Москва
-
Dmitriy Solomnikov » 17.04.2006 (Пн) 18:06
экспорт делается через DLLImport (DllImportAttribute)...
Вернуться в Visual Basic .NET
Кто сейчас на конференции
Сейчас этот форум просматривают: нет зарегистрированных пользователей и гости: 5